Integrating DBLSQD with Electron
With DBLSQD it’s easier than ever to set up auto-updates and update notifications for your Electron app.
dblsqd.autoUpdater
dblsqd.autoUpdater
is
currently compatible with Squirrel.Windows, Squirrel.Mac and AppImage installers.
If you want to use our installer-agnostic update UI, check out the next section. If you want to build a completely customized update process, check out our
Node.js SDK instead.
Electron comes with a basic autoUpdater module that makes use of the Squirrel framework. Using this solution can be complicated and doesn’t provide a very nice user experience
dblsqd.autoUpdater
takes care of updating your Electron application and even adds Linux support.
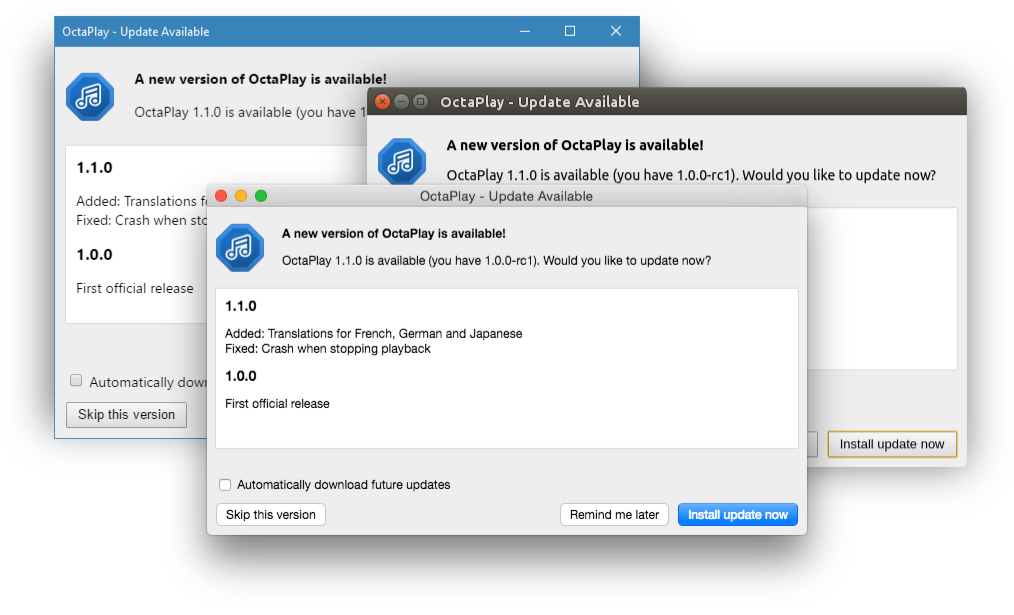
This is what the drop-in UI looks like on macOS, Ubuntu and Windows.
Of course, you easily build your own interface with the SDK.
Installation
You can install dblsqd-electron
with
npm npm install --save dblsqd-electron
or add it to the dependencies in your
package.json
.
Integration
Next, you need to include dblsqd.autoUpdater
in
your application code.
import autoUpdater from "dblsqd-electron"
const updater = new autoUpdater("https://feeds.dblsqd.com/:app_token", "release")
Make sure to include this code as early as possible in your program. Don’t wrap it in an app.on("ready") callback.
Setting up electron-builder (optional)
The easiest way to build an installer for an Electron application is arguably the
electron-builder
package.
If you don’t have it installed yet, also add it to your dependencies:
npm install --save electron-builder electron-builder-squirrel-windows@"^19.42.0"
Later versions of electron-builder-squirrel-windows introduce a bug that can make building the installer fail. This is why we recommend sticking with the specific version suggested above.
Now you need to add the settings for
electron-builder
to your package.json
.
Here is a minimal configuration example for Windows, macOS and Linux:
"build": {
"appId": "com.example.yourapp",
"copyright": "© 1970 Your Corporation",
"win": {
"target": "squirrel"
},
"squirrelWindows": {
"iconUrl": "https://example.com/remote-app-icon.ico",
"remoteReleases": "https://feeds.dblsqd.com/:app_token/:channel/squirrel.windows"
},
"linux": {
"target": "appImage"
}
}
After adding these settings, you can build an installer for the current platform by simply calling
electron-builder
.
Push those artifacts to your DBLSQD store and your updates are live!
You’re now ready to distribute and update your application with DBLSQD!
Using the drop-in UI
Installation
You can install dblsqd-electron
with
npm npm install --save dblsqd-electron
or add it to the dependencies in your
package.json
.
Usage
Here is an example of how to use
dblsqd-electron
:
const {Feed} = require("dblsqd-sdk")
const {UpdateWindow} = require("dblsqd-electron")
let feed = new Feed("https://feeds.dblsqd.com/:app_token", ":channel", ":os", ":arch")
let updateWindow = new UpdateWindow(feed)
Using electron.autoUpdater directly
electron.autoUpdater
does not support update notifications and only works on macOS and Windows. In
most cases, using dblsqd.autoUpdater
, the
drop-in UI or building a custom solution would be a better choice.
DBLSQD supports the feed types of both Squirrel.Mac and Squirrel.Windows, so
all you need to do in order to integrate DBLSQD is to call
setFeedURL()
with the URL of the
corresponding DBLSQD Feed:
const {autoUpdater} = require("electron")
const feedBaseUrl = "https://feeds.dblsqd.com/:app_token/:channel"
switch (process.platform) {
case "darwin":
autoUpdater.setFeedURL(feedBaseUrl + "/mac/x86_64/squirrel.mac")
break
case "win32":
autoUpdater.setFeedURL(feedBaseUrl + "/mac/x86/squirrel.windows")
break
}
Manual Integration
Manually integrating DBLSQD into your Electron application allows for more sophisticated behaviors than the drop-in UI or Electron.autoUpdater.
When manually integrating DBLSQD into an Electron application, we recommend using the DBLSQD node.js SDK to retrieve information about available updates.
Alternatively, you can also directly access the JSON Feed since parsing JSON is very straightforward with JavaScript.